Understanding and Implementing Coroutines in Unity
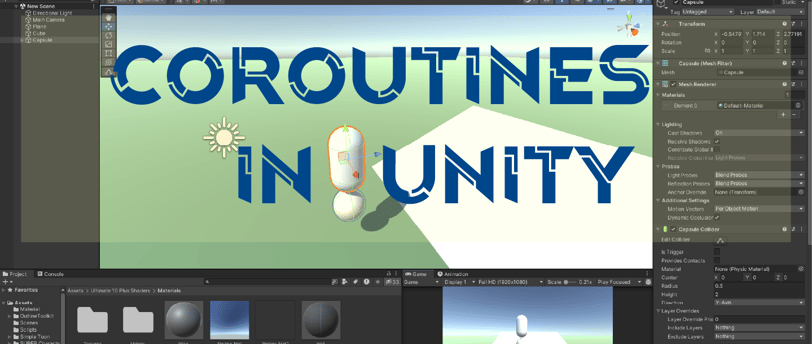
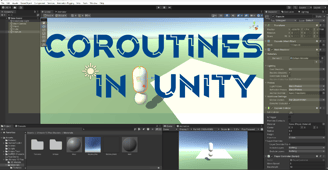
Coroutines are a powerful feature in Unity that allow developers to write asynchronous code that executes over multiple frames. They are essential for tasks such as animations, delays, or any operation that needs to be spread out over time without blocking the main thread. In this blog post, we'll delve into what coroutines are, how they work, and provide examples of their usage.
What are Coroutines? Coroutines in Unity are special functions that can pause execution and yield control back to Unity's game loop. Unlike regular methods, which execute from start to finish in a single frame, coroutines can yield control at specified points and resume execution later. This makes them perfect for tasks that require time-based or asynchronous behavior.
How to Use Coroutines in Unity
Define Coroutine Methods: Create coroutine methods using the IEnumerator return type instead of void.
Start Coroutines: Use StartCoroutine() to begin executing a coroutine method.
Yield Control: Use yield return statements within the coroutine to pause execution and specify when to resume.
Coroutine Execution: Coroutines execute alongside other game logic and are spread out over multiple frames.
Conclusion: Coroutines are a fundamental part of Unity development, providing a way to handle time-based or asynchronous tasks effectively. By understanding how coroutines work and employing them in your projects, you can create smoother animations, implement delays, or manage complex behaviors without blocking the main thread. With the examples provided in this blog post, you should now have a solid foundation for incorporating coroutines into your Unity projects. Happy coding!
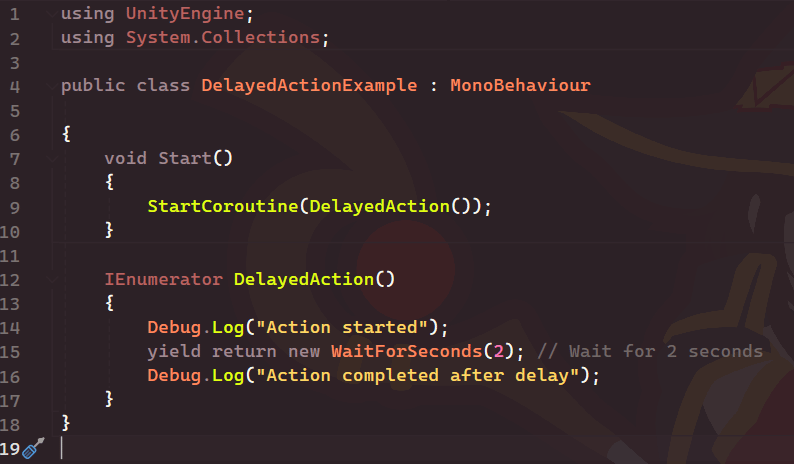
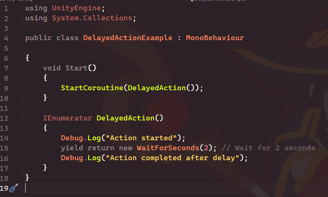
To stop a specific coroutine, you can use the StopCoroutine method. This method can be used with either the coroutine reference or the method name.
When you start a coroutine, you can save the reference to it and then use this reference to stop the coroutine.
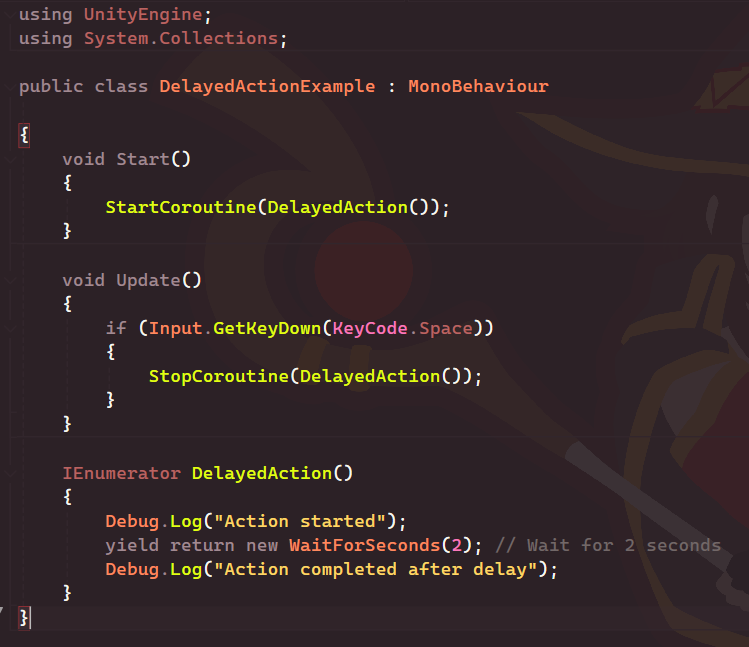
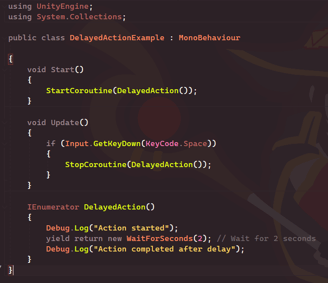
To stop all coroutines running on a particular MonoBehaviour, you can use the StopAllCoroutines method.
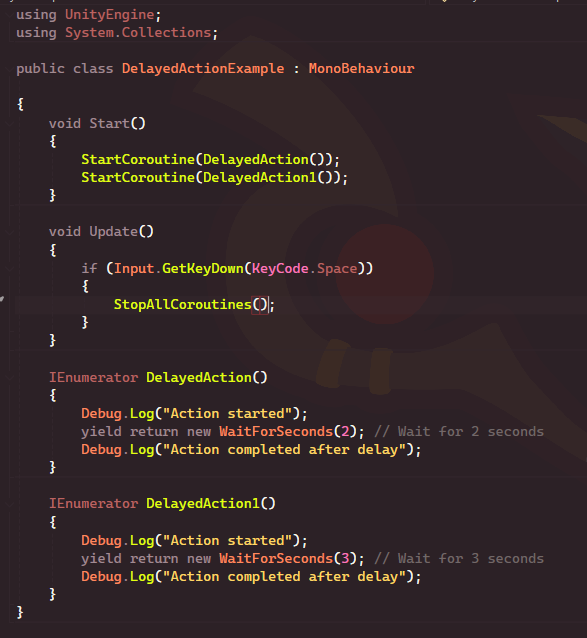
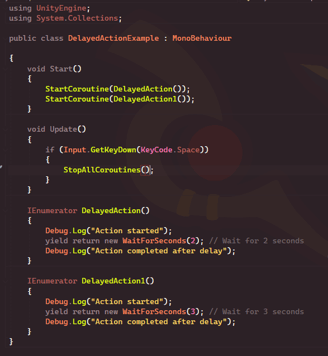